In today’s cross-platform development landscape, handling image conversions efficiently has become increasingly critical.
Converting multi-frame TIFF files to animated GIFs presents unique challenges, especially when working across different operating systems.
This comprehensive guide explores the intricacies of TIFF to GIF conversion in .NET environments, offering practical solutions and performance optimization strategies.
Understanding the TIFF and GIF Formats
The Tagged Image File Format (TIFF) stands as a versatile container for image data, supporting multiple frames and various compression methods.
Unlike simpler formats, TIFF files can store multiple images within a single file, making them ideal for scientific imaging and professional photography.
When working with multi-frame TIFFs in .NET Core, developers must understand the format’s complexity to ensure proper handling.
GIF (Graphics Interchange Format), while older, remains popular for animated content on the web. Its limited color palette of 256 colors per frame might seem restrictive, but this limitation often works in favor of smaller file sizes.
When converting from TIFF to animated GIF, careful consideration must be given to color quantization and frame timing to preserve the original content’s integrity.
Challenges in Converting Multi-Frame TIFF to GIF in Cross-Platform Environments
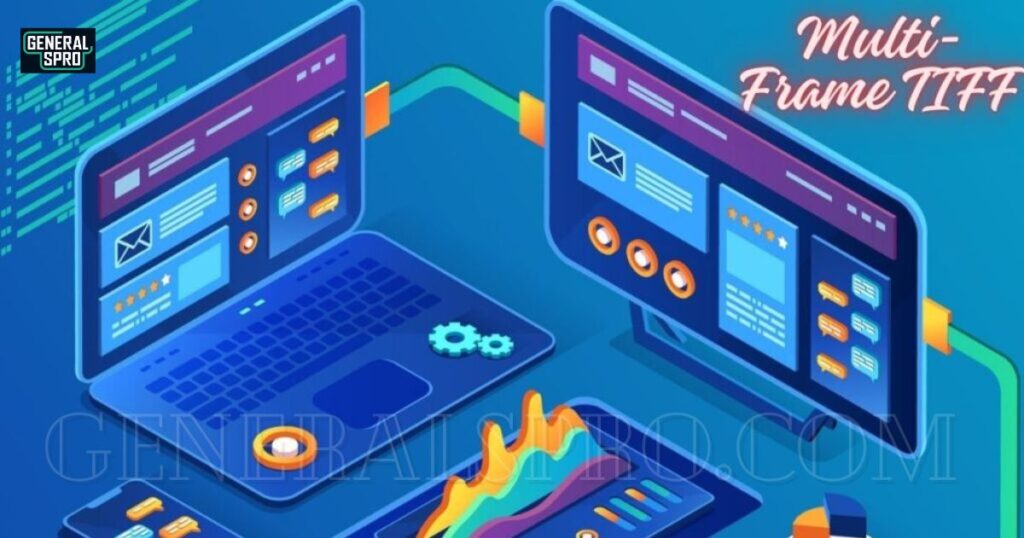
Cross-platform development introduces several complexities when managing multi-frame TIFFs in C#. Memory management varies significantly across operating systems, requiring careful consideration in code implementation.
A key challenge lies in maintaining consistent performance while handling large TIFF files across different platforms.
Common issues developers face include:
- Memory allocation differences between Windows and Unix-based systems
- Varying performance characteristics across platforms
- Color space conversion accuracy
- Frame timing synchronization
- Resource cleanup patterns
Tools and Libraries for Image Conversion
SixLabors.ImageSharp has emerged as a powerful solution for cross-platform image processing in .NET environments. This modern imaging library offers excellent performance characteristics and a clean API for handling multi-frame TIFF files.
Performance comparison for ImageSharp across platforms:
Platform | Memory Usage | Processing Speed | Thread Utilization |
Windows | Moderate | Excellent | Highly Efficient |
Linux | Low | Very Good | Efficient |
macOS | Low | Very Good | Efficient |
Magick.NET
Magick.NET provides comprehensive image manipulation capabilities through its wrapper of ImageMagick.
Its robust feature set makes it particularly suitable for complex TIFF to GIF conversion scenarios.
Converting Multi-Frame TIFF to GIF Using ImageSharp
When implementing TIFF to GIF conversion with ImageSharp, proper resource management becomes crucial. Here’s an example implementation demonstrating efficient GIF creation from TIFF:
Converting Multi-Frame TIFF to GIF Using Magick.NET
Magick.NET offers sophisticated control over the conversion process, particularly useful when dealing with complex TIFF files. The library excels in handling various color spaces and compression methods:
Performance Considerations
When developing cross-platform image conversion tools, performance optimization becomes crucial.
Memory management strategies vary significantly across operating systems, requiring careful consideration in implementation.
Key optimization strategies include:
- Implementing proper disposal patterns
- Utilizing async/await patterns for I/O operations
- Employing memory-efficient processing techniques
- Implementing proper error handling and recovery mechanisms
Best Practices for Error Handling During TIFF to GIF Conversion
Error handling requires special attention when processing multi-frame TIFF files across different platforms. Developers must implement robust exception management strategies that account for platform-specific quirks.
This includes handling file system permissions, memory allocation failures, and corrupt image data gracefully.
A comprehensive error handling approach should include detailed logging, user-friendly error messages, and appropriate fallback mechanisms.
Memory Management Strategies for Large TIFF Files
When converting large multi-frame TIFF files to animated GIFs, memory management becomes crucial for application stability. Implementing proper streaming techniques and buffer management can significantly reduce memory overhead.
Consider using memory-mapped files for large TIFF processing and implementing progressive loading techniques to handle frames in batches rather than loading the entire file into memory at once.
Color Management and Quantization Techniques
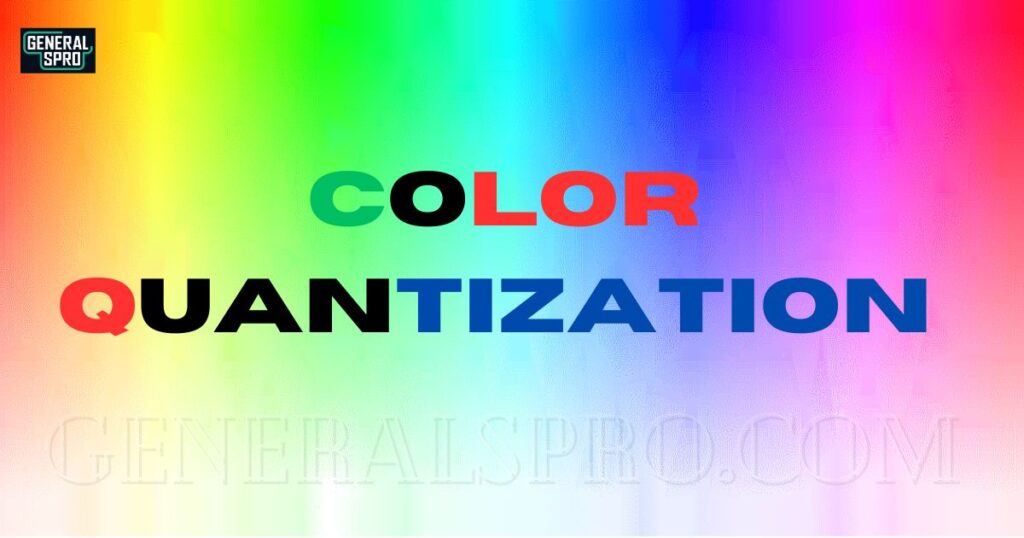
Color management plays a vital role in preserving image quality during TIFF to GIF conversion. Advanced quantization algorithms help maintain color fidelity while adhering to GIF’s 256-color limitation.
Implementing proper color space conversion and dithering techniques ensures optimal visual quality in the resulting animated GIFs.
Cross-Platform File System Considerations
Working with files across different operating systems presents unique challenges. Path separators, file permissions, and naming conventions vary between Windows, Linux, and macOS.
Implementing platform-agnostic file handling routines ensures consistent behavior across different environments while maintaining optimal performance.
Optimization Techniques for Frame Timing
Frame timing optimization is essential for creating smooth animated GIFs from multi-frame TIFFs. Understanding frame delay calculations and implementing proper timing synchronization ensures natural animation playback.
Consider implementing frame skipping algorithms for large TIFF files to maintain reasonable file sizes without sacrificing animation quality.
Integration with Cloud Services
Modern applications often require cloud integration for image processing. Implementing cloud-aware TIFF to GIF conversion services requires careful consideration of bandwidth usage, latency, and scalability.
Consider implementing asynchronous processing queues and progress tracking mechanisms for long-running conversions.
Batch Processing Implementation
Batch processing capabilities are essential for handling multiple TIFF files efficiently. Implementing proper queuing mechanisms and parallel processing strategies can significantly improve throughput. Consider implementing worker pools and progress tracking for large batch operations.
Testing Strategies Across Platforms
Comprehensive testing across different platforms ensures reliable TIFF to GIF conversion. Implementing automated testing pipelines that cover various operating systems, image sizes, and formats helps maintain code quality.
Consider implementing performance benchmarks and quality metrics as part of the testing strategy.
Security Considerations in Image Processing
Security plays a crucial role in image processing applications. Implementing proper input validation, resource limits, and sanitization prevents potential security vulnerabilities.
Consider implementing proper access controls and validation mechanisms for uploaded TIFF files.
Metadata Handling and Preservation
Preserving relevant metadata during TIFF to GIF conversion ensures important image information isn’t lost. Implementing proper metadata extraction and mapping strategies helps maintain image provenance. Consider creating custom metadata storage solutions for information that doesn’t map directly to the GIF format.
Performance Monitoring and Profiling
Implementing comprehensive performance monitoring helps identify bottlenecks and optimization opportunities.
Consider implementing telemetry collection and analysis tools to track conversion performance across different platforms and usage patterns.
Containerization Strategies
Containerizing TIFF to GIF conversion services ensures consistent behavior across different deployment environments.
Implementing proper container configurations and resource limits helps maintain reliable operation. Consider implementing health checks and monitoring for containerized conversion services.
API Design for Cross-Platform Compatibility
Designing platform-agnostic APIs ensures consistent integration across different environments. Implementing proper versioning and documentation strategies helps maintain API stability.
Consider implementing proper rate limiting and quota management for API-based conversion services.
Thread Safety and Concurrency
Implementing thread-safe conversion routines ensures reliable operation in multi-threaded environments.
Proper synchronization and resource sharing mechanisms prevent race conditions and deadlocks. Consider implementing proper thread pool management and work distribution strategies.
Logging and Diagnostics Implementation
Comprehensive logging and diagnostics help troubleshoot issues across different platforms. Implementing structured logging and proper log level management ensures efficient problem resolution.
Consider implementing log aggregation and analysis tools for monitoring conversion operations at scale.
Frequently Asked Questions
What’s the maximum file size limit for TIFF to GIF conversion using ImageSharp?
ImageSharp effectively handles TIFF files up to 2GB on 64-bit systems, though performance may vary based on available memory. For optimal results, consider batch processing larger files in segments.
Can I preserve EXIF data when converting from TIFF to GIF using Magick.NET?
While GIF format has limited metadata support, Magick.NET can preserve basic metadata during conversion. However, specialized TIFF metadata fields will need custom handling or storage in separate files.
How do I maintain color accuracy when converting professional photography TIFF files to GIF?
Implement advanced color quantization algorithms and dithering techniques available in both ImageSharp and Magick.NET. Consider using perceptual color space conversion for optimal visual quality.
What’s the recommended approach for handling large batches of TIFF files in a cross-platform environment?
Implement asynchronous processing with proper memory management and progress tracking. Consider using a queue-based system with proper error handling and retry mechanisms.
How can I ensure consistent frame timing across different platforms when creating animated GIFs?
Use platform-independent timing calculations and implement proper frame delay management. Consider using a standardized timing framework that accounts for system-specific variations.
Conclusion
Successfully converting multi-frame TIFF to GIF in cross-platform .NET environments requires a thorough understanding of both formats and careful consideration of platform-specific challenges.
Whether using ImageSharp or Magick.NET, developers must balance performance requirements with image quality needs.
NET frameworks for image processing continue to evolve, staying updated with the latest best practices and tools remains essential for efficient cross-platform development for image conversion.
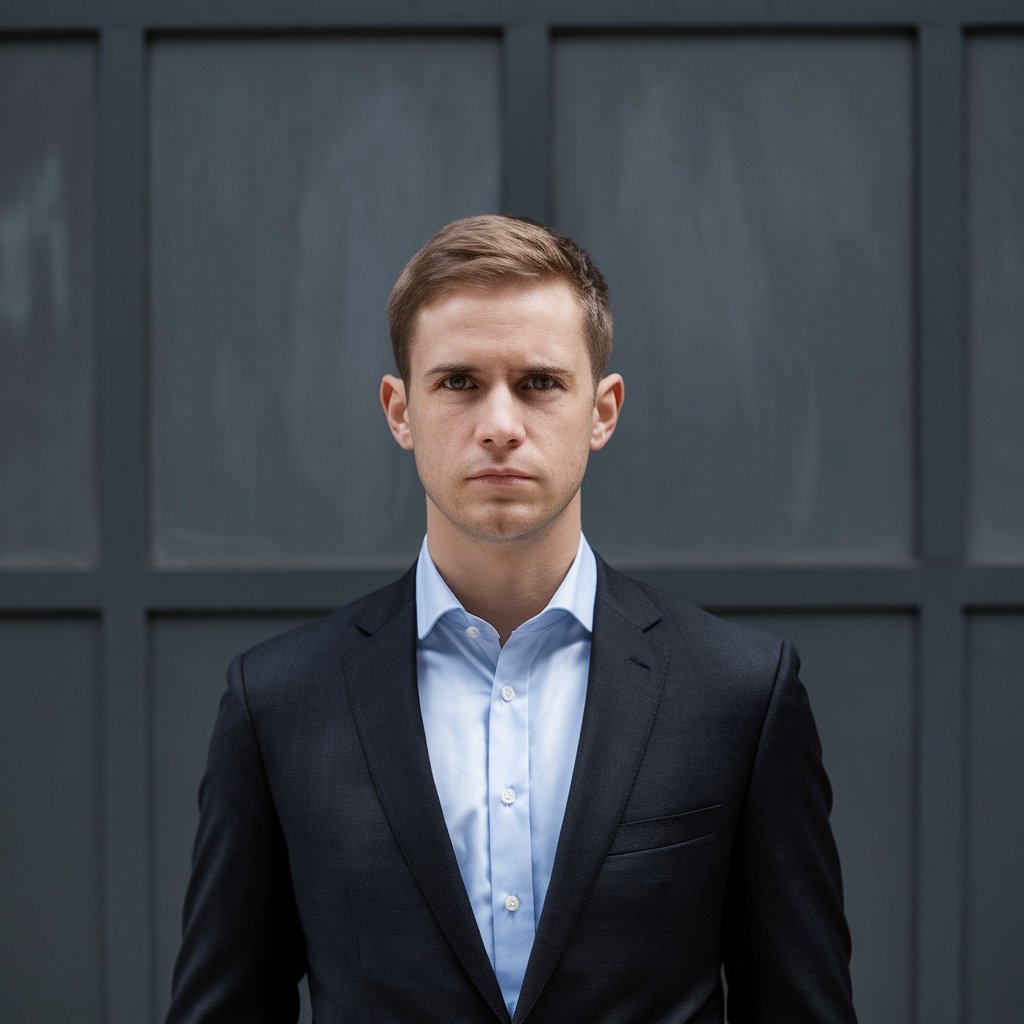